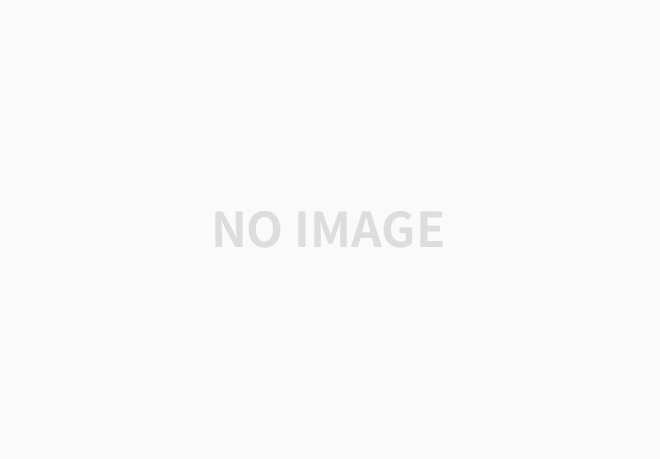
파이어베이스에서 createUserWithEmailAndPassword(auth, email, password)를 사용해 회원가입을 진행한다.
회원 가입에는 email과 password만 입력받도록 되어있다. 이름과 사진 정보를 profile에 저장하려면 가입을 진행한 후 updateProfile()을 사용해야 한다. 프로필사진은 firebase storage에 저장해서 사용하고, 나머지는 string으로 된 정보를 저장하면 된다.
회원가입시 이름, email, password, 프로필사진 정보를 모두 입력받아 저장하기 위해서 아래와 같은 순서로 회원가입을 진행한다.
1. 이름, email, password, 프로필사진 입력받는다
2. email, password로 유저를 만든다.
3. 프로필사진을 firebase storage에 업로드한다.
4. 가입한 유저 정보에 이름, 프로필사진 정보를 저장한다.
1. 이름, email, password, 프로필사진 입력받는다
2-4번이 signin 함수 내에서 순서대로 진행된다.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
import { getAuth, createUserWithEmailAndPassword, updateProfile } from "firebase/auth";
const auth = getAuth();
export const signup = async ({ name, email, password, photo }) => {
// 2. email, password로 유저를 만든다.
const { user } = await createUserWithEmailAndPassword(auth, email, password);
// 3. 프로필사진을 firebase storage에 업로드한다.
const photoURL = await uploadImage(photo);
// 4. 가입한 유저 정보에 이름, 프로필사진 정보를 저장한다.
await updateProfile(auth.currentUser, { displayName: name, photoURL });
return user;
};
|
cs |
2. email, password로 유저를 만든다.
createUserWithEmailAndPassword(auth, email, password)를 통해서는 email, password 정보를 가진 사용자만 만들 수 있다. 신규 계정 생성에 성공하고 나면 사용자가 자동적으로 로그인된다.
자바스크립트를 사용하여 비밀번호 기반 계정으로 Firebase에 인증하기 | Firebase Documentation
의견 보내기 자바스크립트를 사용하여 비밀번호 기반 계정으로 Firebase에 인증하기 Firebase 인증을 사용하여 사용자가 이메일 주소와 비밀번호로 Firebase에 인증하도록 하고, 앱의 비밀번호 기반
firebase.google.com
3. 프로필사진을 firebase storage에 업로드한다.
사진 업로드한 후 사진의 주소 반환하는 함수 uploadImage를 만든다.
1
2
3
4
5
6
7
8
9
|
import { getStorage, ref, uploadBytes, getDownloadURL } from "firebase/storage";
const uploadImage = async (url) => {
// url 확인
if (url.startsWith("https")) {
// http로 시작하는 경우 업로드 필요 없음
return url;
}
}
|
cs |
입력받은 url을 확인해 http로 시작하는 경우는 업로드가 필요 없으므로 그대로 입력받은 url을 반환한다.
프로필 사진을 입력받을 때 imagePicker를 이용해 모바일 기기에 있는 사진을 선택하도록 했으니 로컬 파일의 URI가 전달된다. 그 사진을 blob형태로 가져와서 반환한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
import { getStorage, ref, uploadBytes, getDownloadURL } from "firebase/storage";
const uploadImage = async (url) => {
// url 확인 생략
// 사진 저장 위한 blob 생성
const blob = await new Promise((resolve, reject) => {
// image 불러오기 위한 XML 만든다
const xhr = new XMLHttpRequest();
// imagePicker통해 선택된 사진을 blob형태로 가져온다
xhr.open("GET", url, true);
xhr.responseType = "blob";
// XML 상태 확인
xhr.onload = function () {
// 성공하면 Promise의 값으로 xhr.response 반환
resolve(xhr.response);
};
xhr.onerror = function () {
// 실패하면 Promise의 값으로 Error 반환
reject(new TypeError("Network request failed"));
};
// "GET" 인 경우에는 서버에 데이터를 보낼 필요 없음
xhr.send(null);
});
}
|
cs |
XMLHttpRequest(XHR) 는 서버와 상호작용 하기 위해 만드는 객체이다. 서버에서 모든 종류의 데이터를 받아올 수 있고, 데이터를 서버에 보낼 수도 있다. 사진을 가져오는 과정이니 GET method로 가져오고, 아무것도 보내지 않는다.
( XMLHttpRequest의 속성, 이벤트 등 : https://developer.mozilla.org/ko/docs/Web/API/XMLHttpRequest )
( 비동기작업 위한 Promise 참고 : https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Promise )
( Promise에서 XHR로 이미지 불러오는 예제 : https://github.com/mdn/js-examples/tree/master/promises-test )
user정보 가져와서 user별 폴더에 저장한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
import { getStorage, ref, uploadBytes, getDownloadURL } from "firebase/storage";
// const auth = getAuth();
const uploadImage = async (url) => {
// url 확인 생략
// 사진 저장 위한 blob 생성 생략
// user정보 가져와서 user별 폴더에 저장
const user = auth.currentUser;
const storage = getStorage();
// storage에 업로드 경로 생성
const profileRef = ref(storage, `/profile/${user.uid}/photo.png`);
// blob(사진파일)을 경로에 업로드한다
await uploadBytes(profileRef, blob, {
connectType: "image/png",
});
blob.close();
// 업로드한 사진 주소 반환
return await getDownloadURL(profileRef);
}
|
cs |
성공적으로 계정이 생성되면 자동적으로 로그인되기 때문에 auth.currentUser로 사용자 정보를 가져올 수 있다.
ref를 통해 프로필사진을 업로드할 주소를 가져오고, uploadBytes로 해당 경로에 사진을 업로드한다.
마지막으로 업로드한 사진의 주소를 반환한다.
( uploadBytes : https://firebase.google.com/docs/storage/web/upload-files#upload_from_a_blob_or_file )
4. 가입한 유저 정보에 이름, 프로필사진 정보를 저장한다.
1
2
3
4
5
6
|
export const signup = async ({ name, email, password, photo }) => {
// const { user } = await createUserWithEmailAndPassword(auth, email, password);
// const photoURL = await uploadImage(photo);
await updateProfile(auth.currentUser, { displayName: name, photoURL });
return user;
};
|
cs |
signup은 회원가입, 다른정보까지 업데이트 한 후 생성된 계정 정보를 반환한다.
( uploadProfile : https://firebase.google.com/docs/reference/js/v8/firebase.User#updateprofile )
참고한 페이지
자바스크립트를 사용하여 비밀번호 기반 계정으로 Firebase에 인증하기 | Firebase Documentation
의견 보내기 자바스크립트를 사용하여 비밀번호 기반 계정으로 Firebase에 인증하기 Firebase 인증을 사용하여 사용자가 이메일 주소와 비밀번호로 Firebase에 인증하도록 하고, 앱의 비밀번호 기반
firebase.google.com
https://developer.mozilla.org/ko/docs/Web/API/XMLHttpRequest
XMLHttpRequest - Web API | MDN
XMLHttpRequest 는 이름으로만 봐서는 XML 만 받아올 수 있을 것 같아 보이지만, 모든 종류의 데이터를 받아오는데 사용할 수 있습니다. 또한 HTTP 이외의 프로토콜도 지원합니다(file 과 ftp 포함).
developer.mozilla.org
https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Promise
Promise - JavaScript | MDN
Promise 객체는 비동기 작업이 맞이할 미래의 완료 또는 실패와 그 결과 값을 나타냅니다.
developer.mozilla.org
https://github.com/mdn/js-examples/tree/master/promises-test
GitHub - mdn/js-examples: Code examples that accompany the MDN JavaScript/ECMAScript documentation
Code examples that accompany the MDN JavaScript/ECMAScript documentation - GitHub - mdn/js-examples: Code examples that accompany the MDN JavaScript/ECMAScript documentation
github.com
'React Native' 카테고리의 다른 글
[React Native] navigation (화면설정과 이동) (0) | 2022.02.09 |
---|---|
[React Native] ImagePicker 사용해 사진 업로드하기 (0) | 2022.01.21 |
[react native] 로그인 할 때 이메일 유효성 검사 (0) | 2022.01.18 |
[RN, Firebase] Firebase로 사용자 인증 받기 (0) | 2022.01.17 |
[RN, Firebase] Firebase시작하기, Storage 사용하기 (0) | 2022.01.14 |